【PHP】array_mapと具体的なシチュエーション
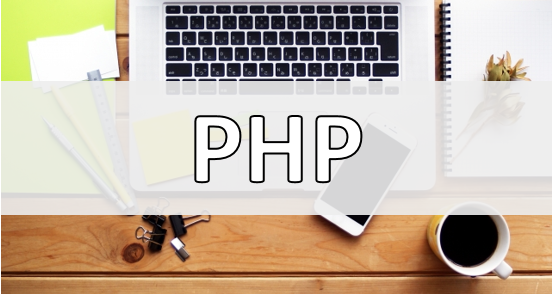
array_mapとは
配列(空、データ有)に対して、加えたい処理(コールバック関数)を適用して、新しい配列を返す。
配列は複数渡せるが、コールバック関数に渡す引数と同数に揃えないと、エラー(ArgumentCountError:引数不足 )になる。

状況
例えば、以下のようなシチュエーションをそれぞれ考えてみる。
- 税率10%をかけた金額を返す。
- 1学年のクラスごとの人数を計算する。
- リクエストされた商品に対して処理を行う。
税率10%をかけた金額を計算する
// 例
$products =
[
[
"id" => 1,
"name" => 'cookie',
"price" => 150,
"type" => 1,
"is_hidden" => 0
],
[
"id" => 2,
"name" => 'ice',
"price" => 200,
"type" => 1,
"is_hidden" => 1
],
[
"id" => 3,
"name" => 'gum',
"price" => 50,
"type" => 2,
"is_hidden" => 0
],
[
"id" => 4,
"name" => 'Potato chips',
"price" => 88,
"type" => 2,
"is_hidden" => 0
],
[
"id" => 5,
"name" => 'candy',
"price" => 30,
"type" => 2,
"is_hidden" => 0
],
];
//float型を整数に
$products = array_map(fn($v)=>[
...$v,
"taxed" => intval($v['price']*1.1)
]
,$products);
1学年のクラスごとの人数を計算する
// 例
$school=
[
[
"id" => 1,
"name" => '1年',
"classes" =>
[
[
"id" => 1,
"name" => '1組',
"students" =>
[
[
"id" => 1,
"name" => 'taro',
],
[
"id" => 2,
"name" => 'kotaro',
],
[
"id" => 3,
"name" => 'aro',
],
[
"id" => 4,
"name" => 'takao',
],
[
"id" => 5,
"name" => 'tataro',
],
],
],
[
"id" => 2,
"name" => '2組',
"students" =>
[
[
"id" => 1,
"name" => 'sato',
],
[
"id" => 2,
"name" => 'ichi',
],
],
],
],
],
[
"id" => 2,
"name" => '2年',
"classes" => [],
],
[
"id" => 3,
"name" => '3年',
"classes" => [],
],
];
function count_students_of_class(array $school): array
{
foreach ($school['classes'] as $class => $students){
$school['classes'][$class]['number_of_students'] = count($students["students"]);
}
return $school;
}
$school = array_map('count_students_of_class', $school);
リクエストされた商品に対して処理を行う
// 例
class Product
{
public function __construct(
int $price,
string $name,
){}
public function create
(
array $product
): array{
$product = array_map(fn($v)=>[
"product_price" => $v['price'],
"product_name" => $v['name'],
],$product);
return $product;
}
}
class ProductCreate
{
public function __construct(
){}
public function create(array $request)
{
$new = new Product($request[0]['price'],$request[0]['name']);
$product = $new->create($request);
var_dump($product);
}
}
$request =
[
[
"price" => 300,
"name" => 'cookie',
],
];
$products = new ProductCreate();
$products->create($request);